Guide to Time Zones and Timestamps
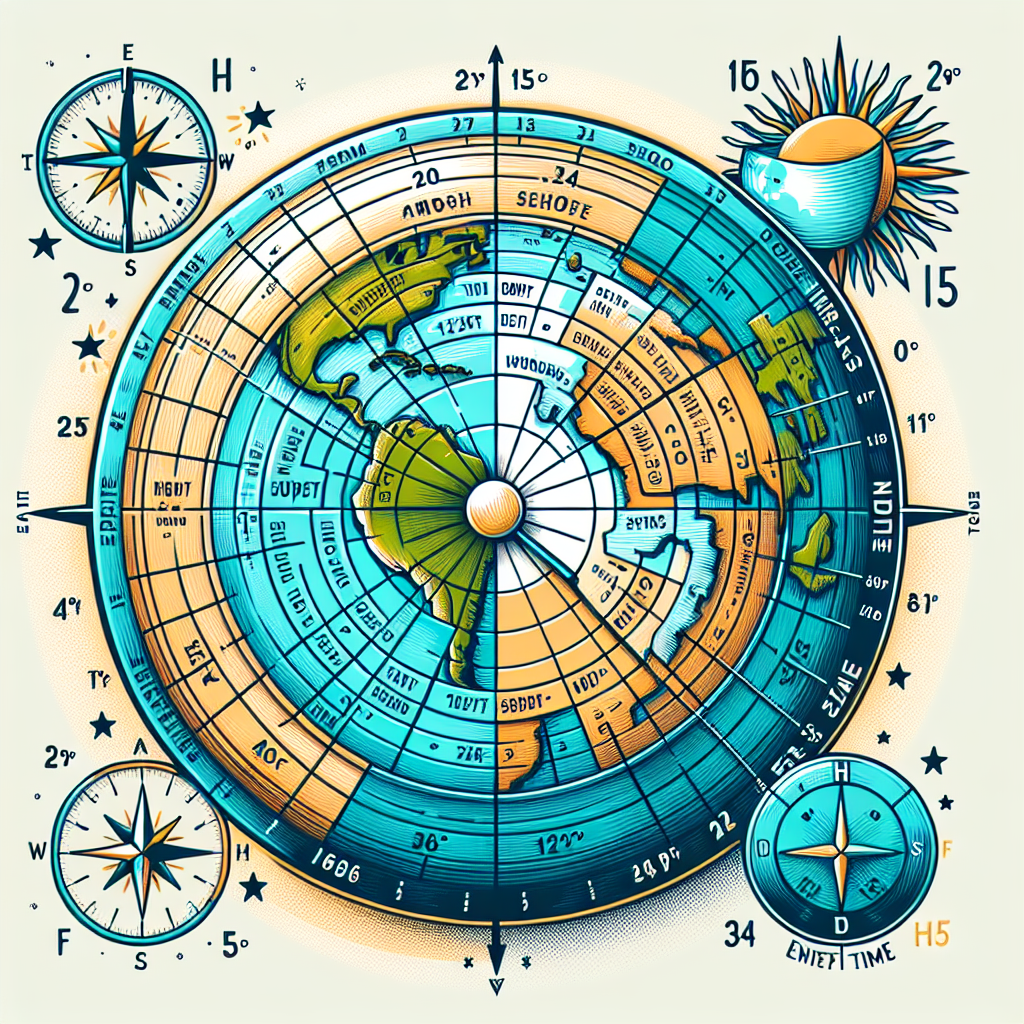
A comprehensive guide to understanding time zones, timestamps, and implementing timezone-aware applications.
Introduction
Ok, before beginning this article, if you are someone who believes that the earth is flat, you can stop reading now. This article won't be suitable for you :)
If you're working with applications that are timezone-dependent and need to handle time across multiple time zones, you've probably encountered some challenges with time zones and timestamps. Since time zones aren't static and change frequently, they can be difficult to work with.
Let's start by understanding what led to this problem and how it originated in the first place.
The Problem
As you know, the Earth is shaped like a sphere, and it rotates around its axis. This rotation causes the sun to rise and set at different times in different parts of the world.
The Earth rotates 360 degrees in 24 hours, which means it rotates 15 degrees per hour. This is why we have 24 time zones, each 15 degrees apart. Each zone differing by one hour from its neighbors.
The problem arises when we need to store and display time in different time zones. We need to store time in a way that allows us to convert it to any timezone we want.
Now you can see that a time zone is simply a longitudinal region of the Earth that observes the same standard time.
Time zones
The concept of time zones is a system designed to standardize time across different regions of the world, facilitating communication and coordination between people in different parts of the world.
According to Wikipedia, the expansion of the system began in the 19th century, when the development of the telegraph and railroads required a standard time system to be used across the world. Sir Sandford Fleming proposed a worldwide system of time zones in 1879. He proposed 24 time zones, each covering 15 degrees of longitude. The system was adopted at the International Meridian Conference in 1884.
You might ask, if there are 24 time zones, each differing by one hour from its neighbors, which time zone do we start from?
The starting point for time zones is called the Prime Meridian. The Prime Meridian is the line of longitude that is 0 degrees. It passes through the Royal Observatory in Greenwich, London, and is also known as the Greenwich Meridian.
Why was Greenwich chosen as the starting point? Because the British Empire was the most powerful empire at that time, with the most ships and trade routes. So, they decided to use Greenwich time as the standard time for the world.
It could have been any other place, but it was Greenwich. Thus, we have the Greenwich Mean Time (GMT) as the standard time reference for the world.
Prime Meridian (0o Longitude)
The starting point for the time zones is called the Prime Meridian. The Prime Meridian is the line of longitude that is 0 degrees. It passes through the Royal Observatory in Greenwich, London, this is the basis coordinated universal time (UTC±0) also known as Greenwich Mean Time (GMT).
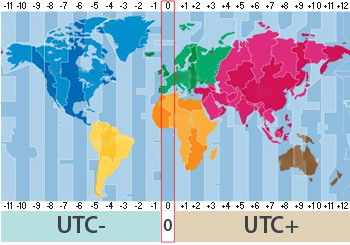
Coordinated Universal Time (UTC) Offset
As you can see from the image above, whenever we move east or west from the Prime Meridian, the time changes by one hour. This is why we have time zones.
Each time zone is expressed as an offset from the Coordinated Universal Time (UTC) ranging from UTC-12 to UTC+14. For example, the time zone of Greenwich is UTC+0, and the time zone of New York is UTC-5.
If you want to see the worldwide time zones, you can check the Worldwide Time Zones list here.
In JavaScript, you can get the current time zone offset in minutes using the getTimezoneOffset()
method of the Date
object.
1const date = new Date();
2const offset = date.getTimezoneOffset();
3console.log(offset); // -120 (UTC+2)
Time Zone Database
The time zone database is a comprehensive collection containing information about all time zones in the world. It includes data about time zone offsets, daylight saving time rules, and time zone names.
The IANA Time Zone Database (also known as the tz database) is the most widely used time zone database. Most operating systems and programming languages rely on it to handle time zones accurately.
In JavaScript, you can use the Intl
object to access time zone information:
1const date = new Date();
2const timeZone = Intl.DateTimeFormat().resolvedOptions().timeZone;
3console.log(timeZone); // Europe/Berlin
For more advanced time zone operations, the moment-timezone
library provides robust functionality in JavaScript applications.
You can find a complete reference of time zone identifiers in the Wikipedia article List of tz database time zones.
Daylight Saving Time
Some regions adjust their clocks during certain parts of the year to extend daylight hours. This practice is known as Daylight Saving Time (DST).
This results in temporary changes to the time zone offset, for example, Egypt switches to Daylight Saving Time (UTC+3) during the summer months (تخفيف احمال).
The rules for DST are not fixed and can change from year to year. This is why it's important to keep your time zone database up to date.
Timestamps
We have 24 time zones, with different offsets. But how do we store time in a way that is timezone-agnostic and allows us to convert it to any timezone we want?
The answer is timestamps.
A timestamp is a digital representation of a specific point in time, often used to record when an event occurred.
A sequence of characters (or numeric value) that makes the exact time of an event.
Examples:
- UNIX timestamp: 1646150400 (seconds since 1970-01-01 00:00:00 UTC)
- ISO 8601 timestamp: 2025-03-01T12:00:00Z (UTC time)
- Human-readable timestamp: March 1, 2025 12:00:00 PM
UNIX Timestamp
The UNIX timestamp is a numeric value representing the number of seconds (or milliseconds) that have elapsed since the Unix epoch (1970-01-01 00:00:00 UTC).
Format:
- Seconds:
1696521600
(October 5, 2023, 00:00:00 UTC). - Milliseconds:
1696521600000
(Commonly used in JavaScript).
Characteristics:
- Always UTC-based (no time zone information).
- Simple Integer value, easy to store and manipulate.
- Negative values are allowed (before 1970).
Handling Time Zones:
- Always UTC-based. To display local time.
- Convert using the local time zone offset. (e.g., UTC+2, adds
7200
seconds). - Example:
1696521600
+7200
=1696528800
(October 5, 2023, 02:00:00 UTC+2).
- Convert using the local time zone offset. (e.g., UTC+2, adds
Usage:
In JavaScript, you can get the current UNIX timestamp using the Date.now()
method which returns the number of milliseconds since the Unix epoch.
const timestamp = Date.now();
console.log(timestamp); // 1646150400000
When working with unix timestamps, you need to know that when you want to show the timestamp as a readable date, you can convert it to a Date
object using the new Date()
constructor. but put in mind that the Date
object will convert the timestamp to your local timezone:
const timestamp = 1646150400000; // Tue Mar 01 2022 16:00:00 GMT+0000
const date = new Date(timestamp);
console.log(date); // 2023-10-05T03:00:00.000Z (UTC+3)
If you want to show the real UTC time, you can add the timezone offset to the timestamp:
const timestamp = 1646150400000; // Tue Mar 01 2022 16:00:00 GMT+0000
const date = new Date(timestamp + new Date().getTimezoneOffset() * 60000);
console.log(date); // 2023-10-05T00:00:00.000Z (UTC)
ISO 8601 Timestamp
The ISO 8601 timestamp is a standardized, human-readable string format for representing dates and times, defined by the International Organization for Standardization (ISO).
Format:
- Basic: 2023-10-05T12:34:56Z (the
Z
indicates UTC time). - With time zone offset: 2023-10-05T12:34:56+02:00 (UTC+2 time).
- With milliseconds:
2023-10-05T12:34:56.789Z
.
Characteristics:
- Self-descriptive format (includes date, time, and time zone).
- Supports time zone via offset or abbreviation. (e.g.,
+02:00
,-05:00
,Z
). - Commonly used in APIs and data interchange formats.
- Flexible precision (year, month, day, hour, minute, second, millisecond, nanosecond).
Handling Time Zones:
- Explicitly includes the offset or uses the
Z
suffix for UTC time. - Example Conversions:
2023-10-05T12:00:00Z
(UTC) →2023-10-05T14:00:00+02:00
(Cairo).2023-10-05T08:00:00-04:00
(New York) →2023-10-05T12:00:00Z
(UTC).
Usage:
In JavaScript, you can get the current ISO 8601 timestamp using the toISOString()
method of the Date
object.
const date = new Date();
const isoTimestamp = date.toISOString();
console.log(isoTimestamp); // 2025-03-01T12:00:00.000Z
Key Differences between UNIX and ISO 8601 Timestamps
Aspect | Unix Timestamp | ISO 8601 Date |
---|---|---|
Format | Integer (Seconds or milliseconds since epoch) | String (YYYY-MM-DDTHH:MM:SSZ) |
Time Zone | Always UTC (no explicit offset). | Can include UTC (Z) or local offsets (±HH:MM). |
Human Readability | Requires conversion to be understood. | Human-readable format, self-descriptive. |
Precision | Seconds or milliseconds. | Flexible (year, month, day, hour, minute, second, millisecond, nanosecond). |
Leap Seconds | Does not account for leap seconds. | Can technically represent 23:59:60 during leap seconds, but rarely used. |
Portability | Requires knowledge of the Unix epoch. | Self-contained (no epoch reference needed). |
Storage Efficiency | Compact (4 or 8 bytes as an integer). | Longer (e.g., 24+ characters as a string). |
Use Cases | System, programming, and storage operations. | APIs, data interchange, human-readable formats. |
Fun Facts
- France has 12 time zones, due to its overseas territories.
- China, despite spanning five geographical time zones, uses a single time zone (UTC+8) for the entire country.
- The International Date Line (IDL) is an imaginary line that runs from the North Pole to the South Pole, roughly along the 180th meridian. Crossing the IDL results in a day change.
- In 2011, Samoa skipped December 30th to switch from the eastern side of the IDL to the western side, aligning its time zone with Australia and New Zealand.
- India has a single time zone (UTC+5:30) for the entire country, despite spanning two geographical time zones.
- The time zone of Nepal is UTC+5:45, which is 15 minutes ahead of India.
- There are ~38 time zones globally due to fractional offsets.
Conclusion
Working with time zones and timestamps is unavoidable in modern web development. By understanding the fundamentals—from the historical Prime Meridian to modern timestamp formats—you can make better decisions about how to handle time in your applications.
For most applications, best practices include:
- Always store timestamps in UTC (either as UNIX timestamps or ISO 8601 format)
- Convert to local time only for display purposes
- Use established libraries like
date-fns
,luxon
, orTemporal
(the upcoming JavaScript API) for complex time operations - Keep your time zone database updated to handle DST changes correctly
Remember that time-related bugs can be subtle and difficult to track down. Taking the time to implement proper time handling from the beginning will save you from late-night debugging sessions trying to figure out why your application is an hour off in certain parts of the world.
As browsers continue to improve their built-in date handling capabilities, working with time zones should become easier—but understanding these fundamentals will always remain valuable knowledge for any developer building global applications.